ACME Mobile Meditation Co
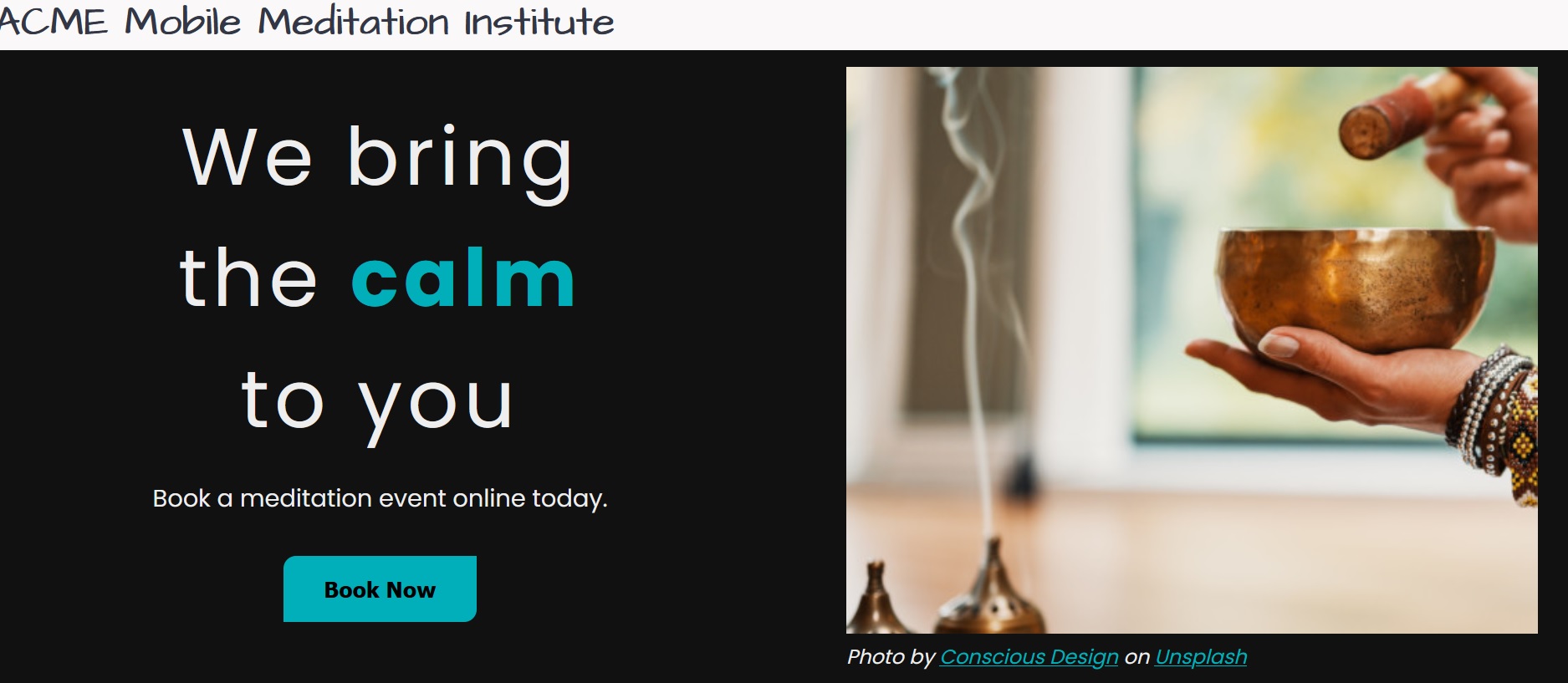
React app which displays home, about, and contact pages for a fictional meditation events business. Inspired by following along with developedbyed in his Creative React and Redux course.
This app uses styled components for style, react-router-dom for page routing, and Framer Motion for animations.
Styled Components⌗
To use styled components with the styled
keyword, first styled-components
must be installed (using npm
or yarn
).
Next, styled components were created and saved to a component using 1 of 2 methods:
- Using
styled.div
,styled.button
, or similar to create styled HTML elements. - Inheriting from another styled component, such as
styled(Section)
.
The Sass-like styling rules for a styled component get wrapped in backticks, ```, and may use nesting.
Here’s an example of creating a new styled component called Work
which inherits from a custom Section
styled component.
OurWork.jsx⌗
Note that the styled component is located outside of the main component.
What I added beyond the tutorial⌗
The focus of this project was to use Framer Motion to add page and component animations. The thing about animations is that they may not be accessible for some people, so I took the opportunity to do more research about making animations accessible.
prefers-reduced-motion⌗
There is a CSS media query called prefers-reduced-motion which can detect whether the user’s browser settings requests the page to minimize motion. This is essential for zoom-type animatons such as pulse or transform, which can be migraine triggers.
The OurWork
page has a rainbow animation that shuffles across the screen. Although it is not a flashing or zooming type of animation, I figured that it would be better to leave off if the user has requested reduced motion. For this reason, I added a display: none
property on the prefers-reduced-motion
query.
useReducedMotion Hook⌗
Framer Motion has a reduced motion hook which can be used in conditions to determine what types of animations to use; for example, replacing transform
animations with opacity animations.